Operators in PHP are symbols that let you manipulate data, perform calculations, make comparisons, and handle logical operations. This guide breaks down the different types of operators, such as arithmetic, assignment, comparison, logical, string, and array operators, to help you write better PHP code.
Key Takeaways
PHP operators are categorised into arithmetic, assignment, comparison, logical, string, and array operators, each serving distinct purposes for data manipulation.
Understanding operator precedence and associativity is crucial for predicting the evaluation order in expressions, enhancing code clarity.
The spaceship operator (<=>) simplifies comparisons between expressions, returning -1, 0, or 1 based on their relative values, and is particularly useful in sorting algorithms.
Understanding PHP Operators
PHP operators are symbols that perform operations on variables and values, making them an essential part of the language. Their primary role is to manipulate data, whether it's for calculations, value assignments, comparisons, or logical expressions. These operators provide efficient and straightforward data manipulation methods, enhancing coding practices and improving code readability.
PHP categorises its operators into several groups: arithmetic, assignment, comparison, logical, string, and array. Each group of operators serves a unique purpose, allowing developers to perform specific tasks efficiently. Understanding these categories will help you write more intuitive and maintainable code using a PHP processor.
Arithmetic Operators in PHP
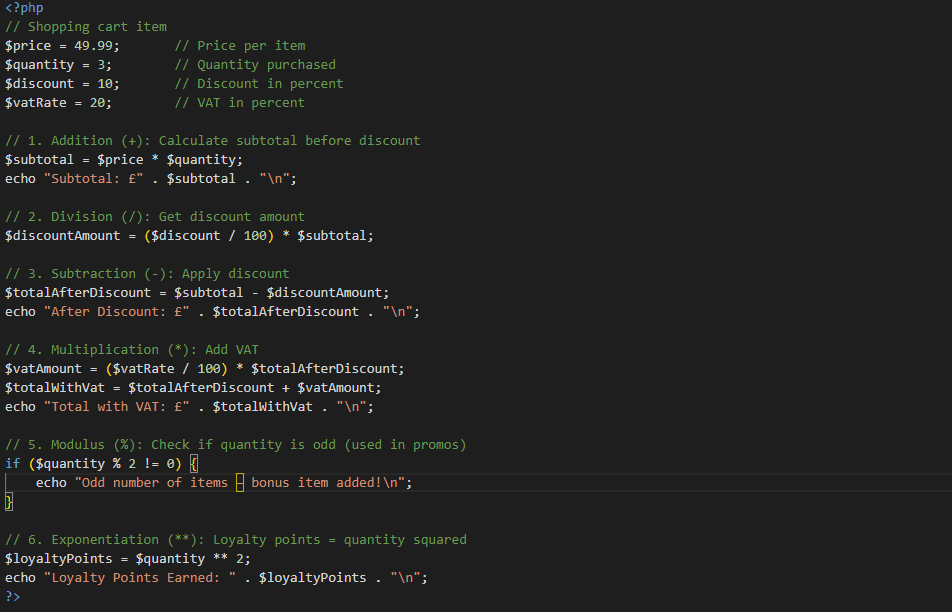
In PHP, arithmetic operators perform common arithmetic operations. They work specifically with numeric values. These operators include:
Addition (+)
Subtraction (-)
Multiplication (*)
Division (/)
Modulus (%). They allow developers to perform mathematical calculations essential for various applications.
The addition operator (+) sums two values, while the subtraction operator (-) finds the difference between them. Arithmetic operators are used to multiply and divide values, respectively. The modulus operator (%) returns the remainder of a division operation involving two operands, which can be particularly useful in exercises, including integer division. Additionally, the division operator can be combined with two operators to perform more complex calculations, as detailed in the operator name description.
These operators are:
Addition (+): sums two values
Subtraction (-): finds the difference between two values
Multiplication (*): multiplies values
Division (/): divides values
Modulus (%): returns the remainder of a division operation and can perform everyday arithmetical operations.
PHP also supports the exponentiation operator (**), which raises a number to the power of another. This operator is handy for more complex mathematical operations, such as calculating powers and roots. Understanding these operators and their usage is crucial for performing efficient calculations in your PHP code.
Assignment Operators Explained
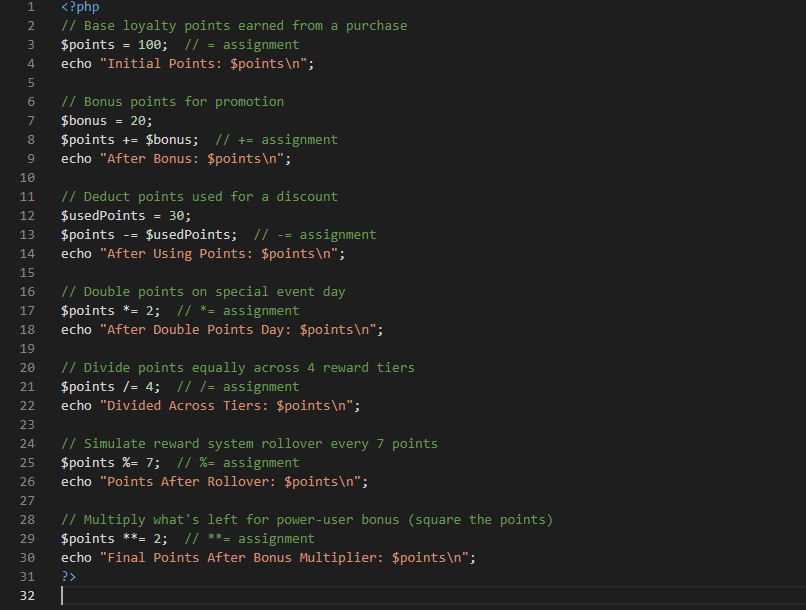
In PHP, assignment operators assign values to variables. The most basic assignment operator is the equals sign (=), which assigns the value on the right to the variable on the left. For example, $a = 5 assigns the value 5 to the variable $a.
There are two types of assignment operators in PHP: simple and compound assignment operators. Simple assignment operators include the basic equals sign, while compound assignment operators combine arithmetic operations with assignment. Examples include +=, -=, *=, and /=, which add, subtract, multiply, and divide a value with the variable, respectively.
Using compound assignment operators can make your code more concise and efficient. For instance, $a += 5 adds 5 to the current value of $a and then assigns the result to $a in a single step. PHP also provides bitwise and logical assignment operators for performing operations based on binary values and rational conditions.
Comparison Operators in Action
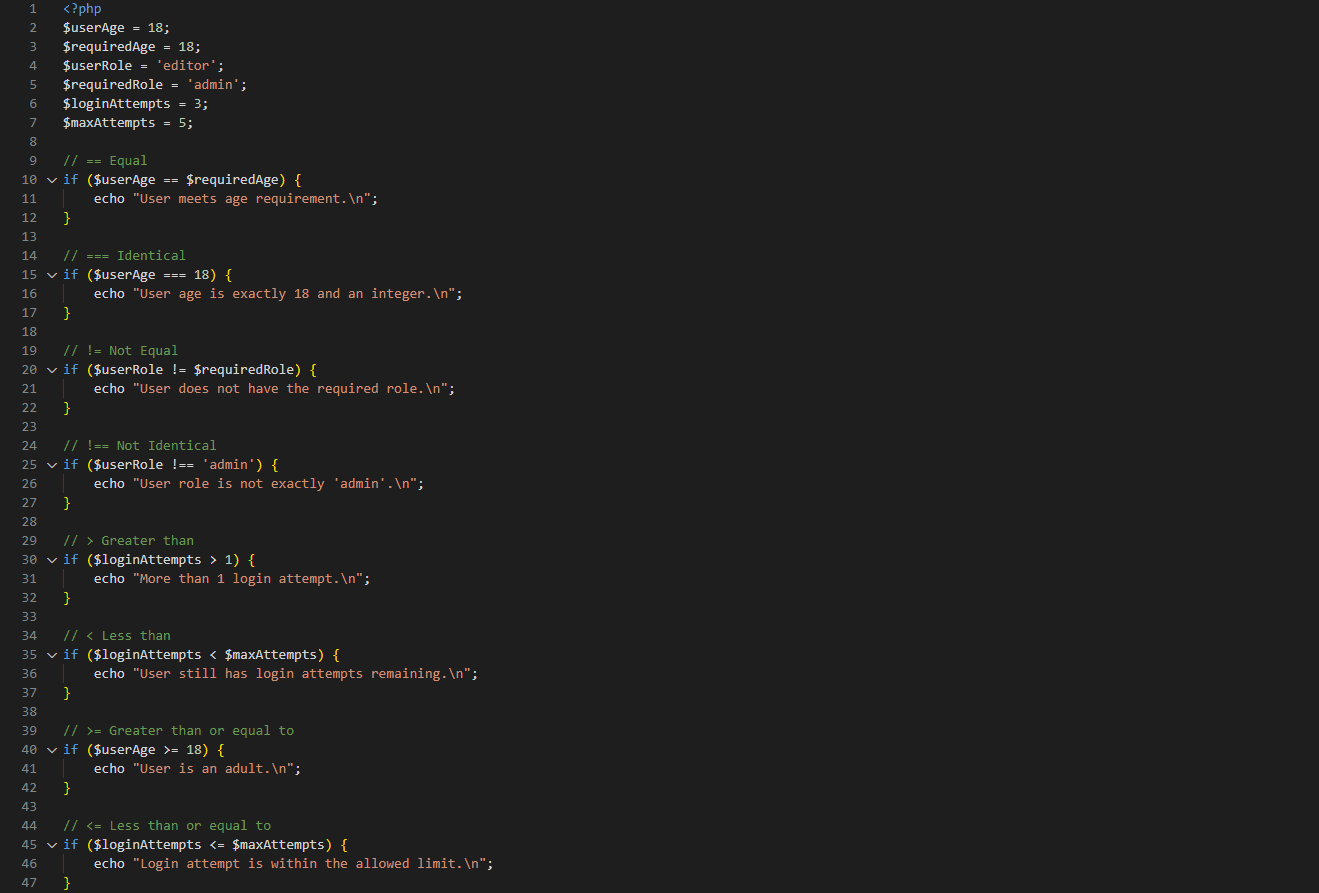
Comparison operators in PHP compare two values and return either true or false Boolean results. These operators are essential for making decisions in your code based on the relationships between values. For example, comparison operators check if two values are equal, while the strict equality operator (===) verifies that they are equal and of the same data type.
Relational comparison operators include the less than (<), greater than (>), less than or equal to (<=), and greater than or equal to (>=) operators. These operators allow you to compare numeric values and determine their relationships. For instance, $a < $b returns true if $a is less than $b, while $a >= $b returns true if $a is greater than or equal to $b.
Using comparison operators effectively enables you to control the flow of your program based on specific conditions. Understanding these operators is crucial for writing robust PHP code, whether comparing user input, checking conditions, or validating data.
Using Increment and Decrement Operators
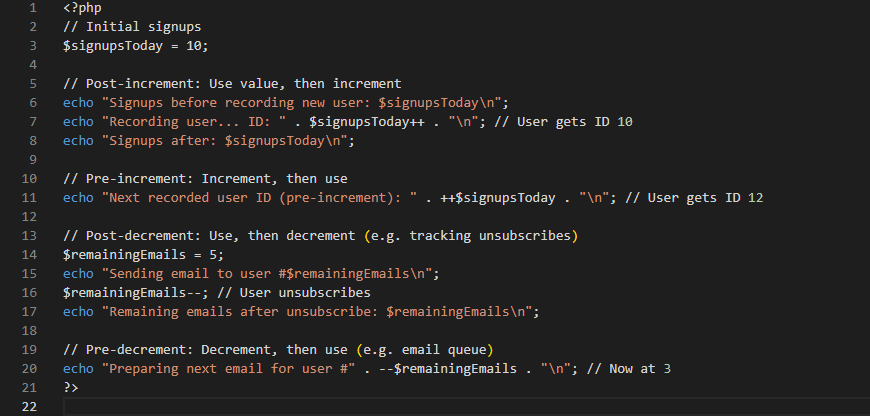
Increment and decrement operators are essential for modifying variable values in simple expressions. The increment operator (++) increases a variable's value by one, while the decrement operator (–) decreases it by one. These increment and decrement operators are handy in loops and iterations, where you need to update the value of a counter or index.
For example, $a++ increases the value of $a by one, while $a– decreases it by one. These operators can be used in prefix and postfix forms, determining when the increment or decrement operation is applied. Understanding these forms' differences and practical applications can help you write more efficient and readable code.
Logical Operators for Conditional Statements
Logical operators in PHP are used to combine conditional statements and control the flow of your program. These operators return Boolean values, either true or false, based on evaluating one or more conditions. The primary logical operators in PHP are AND (&&), OR (||), and NOT (!), each serving a unique purpose in conditional expressions.
The logical operators work as follows:
The AND operator (&&) evaluates to true only if both operands are true. For example, $a && $b
returns true if both $a
and $b
are true.
The OR operator (||) returns true if at least one of the operands is true.
For instance, $a || $b returns true if either $a or $b is true.The NOT operator (!) reverses the truth value of its operand, making actual conditions false and vice versa.
Logical operators are vital for creating complex conditional statements and controlling program flow based on multiple conditions. They can be used in if statements, loops, and other control structures to evaluate conditions and make decisions based on user input or other factors.
String Operators for Concatenation
String operators in PHP are used to manipulate and combine strings. The concatenation operator (.) is particularly important because:
It allows you to join two strings together.
For example, $c = $a. $b merges the strings $a and $b into a single string.
If $a is 'Hello' and $b is 'World!', the result of $c = $a. $b will be 'HelloWorld!'.
This operator is essential for creating dynamic strings and combining text from different sources. The concatenation assignment operator ( .= ) is another helpful tool, which appends the right-hand string to the existing left-hand string. For instance, $a .= $b adds the content of $b to the end of $a, modifying the value of $a in the process. The string operator plays a crucial role in these operations.
Understanding these string operators and their usage can help you create and manipulate strings more effectively in your PHP code.
Array Operators in PHP
Array operators in PHP facilitate comparison between arrays and enable various operations, such as merging and checking equality. The union operator (+) combines two arrays, using the values from the first array when keys overlap. This operator is useful for merging arrays while preserving unique keys.
The equality operator (==) checks if two arrays have identical key/value pairs, regardless of their order. In contrast, the identity operator (===) verifies that two arrays have the identical key/value pairs and maintain the same order. These operators allow you to compare arrays and determine their relationships based on key/value pairs.
The inequality operator (!= or <>) determines if two arrays are unequal regarding key/value pairs. The non-identity operator (!==) checks if two arrays are not identical, differing by key order or value types. Understanding these array operators is crucial for working with PHP arrays effectively.
Conditional (Ternary) Operators
The conditional operator in PHP, known as the ternary operator, is a shorthand method for writing if-else statements. It evaluates an expression for true or false conditions and executes one of two statements based on the result. The syntax consists of:
A condition followed by a question mark (?)
The value is true
A colon (:)
The value is false.
For example, $result = $a > $b ? 'a is greater': 'b is greater'
assigns 'a is greater' to $result
if $a
is greater than $b
; otherwise, it assigns 'b is greater'. This operator can improve code readability by reducing the code needed for simple conditional assignments.
However, nesting ternary operators to handle multiple conditions can reduce readability. Thus, it is essential to use them judiciously and consider alternative methods for more complex conditions.
Operator Precedence and Associativity
Operator precedence in PHP defines how tightly expressions are bound together, affecting their evaluation order. Operators with higher precedence are evaluated before those with lower precedence. For example, multiplication (*) and division (/) have higher precedence than addition (+) and subtraction (-), so they are evaluated first in an expression.
Associativity determines how operators of the same precedence are grouped when evaluating an expression. For instance, the addition and subtraction operators are left-associative, meaning they are evaluated from left to right, with the left operand being processed first. Understanding associativity helps you predict the outcome of expressions with multiple operators.
You can control the order of operations in PHP expressions by using parentheses. Parentheses can enhance the clarity of expressions, making the intended order of operations explicit and easier to read.
The Spaceship Operator (<=>)
The spaceship operator (<=>) was introduced in PHP 7. It is used to compare two expressions. Also known as the combined comparison operator, it returns:
-1 if the first expression is less than the second
0 if the first expression is equal to the second
1 if the first expression is greater than the second
For example, $result = $a <=> $b assigns -1 to $result if $a is less than $b, zero if $a is equal to $b, and one if $a is greater than $b. This operator simplifies comparisons and makes the code more readable by indicating the intent of comparison in conditional expressions.
The spaceship operator is particularly useful in sorting algorithms, where it facilitates custom comparison logic and improves code clarity.
Practical Examples of PHP Operators
Operators are used in PHP for basic data manipulation and executing complex functions in applications. For instance:
Arithmetic operators can be used to perform calculations.
Assignment operators are essential for initialising variables and updating values.
Chained assignment allows multiple variables to be assigned the same value in a single expression, enhancing code efficiency.
Logical operators can control the flow of execution in loops and conditional statements. For example, combining conditions with logical operators can determine user discount eligibility based on multiple criteria. The spaceship operator simplifies comparison logic, making it easier to write custom sorting functions using a bitwise operator and binary operators.
Using these operators effectively can lead to cleaner and more efficient code, improving both performance and readability of your PHP applications.
Exercises to Test Your Knowledge
To test your understanding of PHP operators, try the following exercises:
This exercise helps reinforce the concept of using assignment operators, arithmetic operators, and assigning values to variables.
Next, use comparison operators to check the relationships between two variables. You can combine logical operators for this task. It will help you create complex conditional statements. These exercises will help you apply what you've learned and improve your coding skills.
Summary
In summary, PHP operators are essential tools that enable you to manipulate data, perform calculations, assign values, and make decisions in your code. We have explored various types of operators, including arithmetic, assignment, comparison, logical, string, array, and conditional operators. Each type serves a unique purpose and is crucial for writing efficient, readable PHP code.
Understanding and mastering these operators will enhance your coding skills and enable you to write more robust and maintainable applications. Remember to use operators judiciously and consider their precedence and associativity to ensure your expressions are evaluated as intended. With practice, you'll find that these operators become second nature, making your programming tasks more intuitive and enjoyable.
Frequently Asked Questions
What is the purpose of PHP operators?
PHP operators perform operations on variables and values, enabling data manipulation, calculations, value assignments, and logical comparisons. This functionality significantly enhances the practicality and clarity of your code.
How do arithmetic operators in PHP work?
Arithmetic operators in PHP facilitate mathematical operations such as addition, subtraction, multiplication, division, and modulus on numeric values, enabling practical calculations. Their proper use is essential for performing arithmetic tasks within PHP scripts.
What is the difference between the equality operator (==) and the strict equality operator (===) in PHP?
The equality operator (==) performs type conversion to compare two values, while the strict equality operator (===) requires both the value and the data type to be the same. This distinction is crucial for accurate comparisons in PHP.
How does the ternary operator improve code readability?
The ternary operator enhances code readability by offering a concise way to implement simple conditional assignments. It reduces clutter and clarifies the programmer's intentions. Its use can significantly streamline your code.
What is the spaceship operator (<=>) used for in PHP?
The spaceship operator (<=>) in PHP is utilised for comparing two expressions, returning -1, 0, or 1 based on whether the first expression is less than, equal to, or greater than the second. This operator enhances the efficiency of comparisons, especially in sorting contexts.